You can optimize your bundle size by simply importing manually the components you need.
I’m pretty sure there’s a better way to do this in svelte, since it’s a compiler and you can implement some tree shaking precompiling callback in rollup or webpack.
I’m using rollup in my projects, instead of webpack and here’s how I do it: https://github.com/tncrazvan/svelte-framework7-template
The important pieces are src/framework7-custom.js and src/style/framework7-custom.less.
The js file contains the javascript implementations of the components and the less file contains the links to each component style.
I made sure to implement all components in those files, although they are all commented out.
All you have to do when you want to use a new component, that is not available in your application, is remove the comments from those files for your specific component.
For example, you may want to use the Popup component:
- You’ll have to look for the Popup import in your custom js and remove the comment
- Then look for the Framework7.use(…) call and remove the comment for your Popup component
- Now switch to you custom less file and remove the comment for your component style import
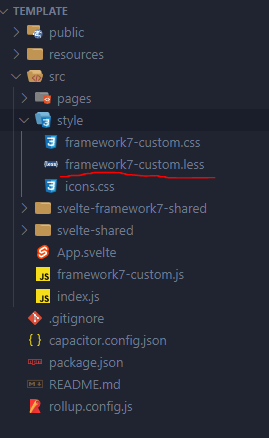
- Compile the less file to css (or use a Svelte less preprocessor) and import the styles in the global scope.
I’m using a VSCode extension called “Easy LESS”, it’s pretty popular, it compiles your less file to a css when you save it (ctrl+s), it uses the same filename as the original except for the extension ofc.
https://marketplace.visualstudio.com/items?itemName=mrcrowl.easy-less
So you’ll get a file like this:
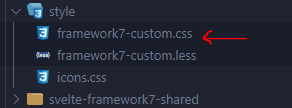
And you can import it into svelte (the template already does it for you):
Now you can use the Popup component, import the component as you would normaly do
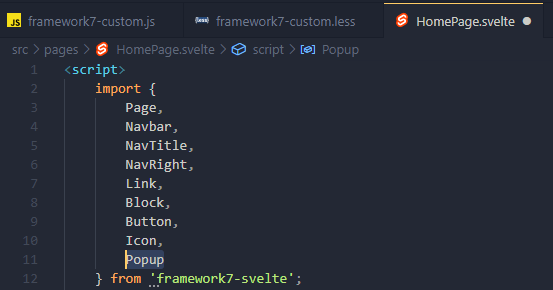
and use it
Output:
Hope this helps!
EDIT:
These are the bundles I’m getting when I run “npm run build” for this specific example:

the css bundle as you can see is very small.
Also you shouldn’t count your “.map” files as part of your application’s weight, those only get downloaded by the browser when you inspect your source code.
BTW, the reason I’m using rollup is because, unlike webpack, it correctly shows the svelte source code:
EDIT 2:
Just to be clear, the “extra.css” file is not needed, that file is not even processed by svelte, it’s a dummy file I made (which is not even minimized) that contains a bunch of icons that framework7 already has anyway.
So all the css your app would download is that 5KB file.
As for the js file, I’m afraid that’s the best we can do, I believe that’s the framework7-dom we’re looking at with 285KB (probably 7KB of those is svelte).